Here is a step by step explanation on how to connect SQL server database with C#. I think there is no nee
d for much explanation since the images are self explanatory.
Our aim is to create a very simple C# application which save an employee name and an employee email ID into SQL server database.
Create DB to store Values
If you don't have Sql Server Management Studio Express , click here to download it
Step 2 : Create a table "tbl_Employee" and create two columns to store Name and Email ID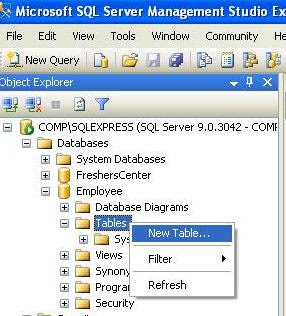
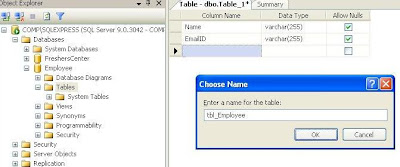
Create a C# application
Step 1 : Open Microsoft Visual Studio 2008 and create a new Project
Step2 : In the Default.aspx Page Create an interface to enter values
It should include the following :
1 Text box to enter Name (txtName)
2. Text box to enter Email ID (txtEmail)
3. A button to save the data.(btnSave)
Step 3 : Specify the connection string value in web.config. Open your web.config file . Search for the following tag $ltconnectionstrings> .
<connectionStrings>
<connectionStrings>
<add name="connString" connectionString="server=COMP\SQLEXPRESS;database=Employee;Integrated Security=SSPI; "/>
</connectionStrings>
If you are not sure about your server name , Open Microsoft Sql Server Management Studio Express and check the server name. See the image below.
Step 4 : Write the code to save the Value to DB in Click event of the Save button.
protected void btnSave_Click(object sender, EventArgs e)
{
string connectionString = ConfigurationManager.ConnectionStrings["connString"].ConnectionString;
SqlConnection cnn = new SqlConnection();
cnn.ConnectionString = connectionString;
SqlCommand sqlCommand = new SqlCommand();
sqlCommand.Connection = cnn;
cnn.Open();
try {
sqlCommand.CommandText = "INSERT INTO tbl_Employee ([Name],[EmailID]) VALUES " + "('" + txtName.Text + "','" + txtEmail.Text + "')";
sqlCommand.ExecuteNonQuery();
lblStatusMessage.Text = "Data inserted successfully";
}
catch (Exception ex)
{
Response.Write("An error occurred while inserting values to DB
" + ex.ToString());
}
finally {
if (cnn.State == ConnectionState.Open) cnn.Close();
}
}
Now you are ready to go.. Run the application.